Overview
This tutorial will teach you how to setup a DC motor with the Satellite boards built-in Mosfet and how to control the motor speed using Analog input.
Components
Arduino
A/B USB connectors
Museduino Shield
2 Satellite boards
CAT5 cable
DC Motor
10k Potentiometer
screw driver
Setup
First, complete the instructions for the DC Motor tutorial.
Now let’s add potentiometer on Arduino Analog Pin A0 using a second Satellite board. Use the Analog Select chart to configure the jumper shunts in the correct Down position.
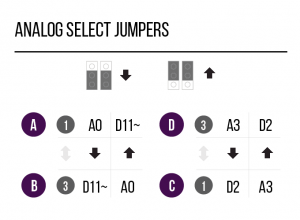
Once you’ve configured the jumpers, we need to wire the potentiometer to the following screw terminal configuration:
1 – Power
2 – Direct Signal
4 – Ground
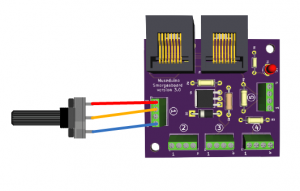
Each Satellite is designed with 2 RJ-45 connectors allowing multiple Satellite boards to be daisy chained with distance between sensors/actuators.
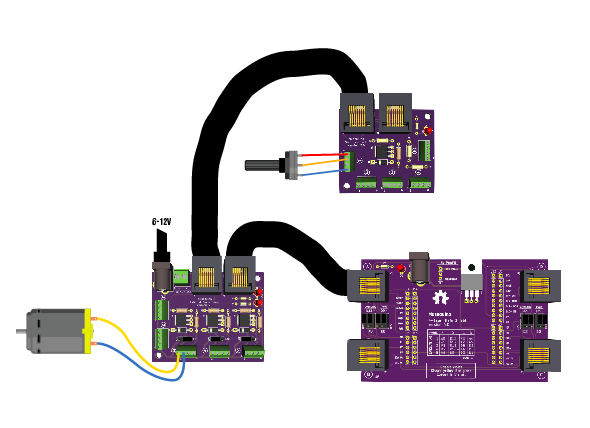
Code
Copy code below or download from Github. Upload the sketch.
/*
Museduino | DC Motor Tutorial
Control the speed of a DC moto with a potentiometer
*/
//satellite Pin 1 on Port A
int s1A = A0;
//satellite Pin 3 on Port A
int s3A = 9; //default arduino pin is D8, use Analog Select to swap with D9~
int motorSpeed = 0; //motor speed value
// the setup routine runs once when you press reset:
void setup() {
Serial.begin(9600);
// initialize potentiometer as input and the motor pin as an output.
pinMode(s1A, INPUT);
pinMode(s3A, OUTPUT);
}
// the loop routine runs over and over again forever:
void loop() {
//read value from potentiometer
motorSpeed = analogRead(s1A);
//map value from 0 (off) to 255 (on)
motorSpeed = map(motorSpeed, 1023, 0, 0, 255);
Serial.println(motorSpeed);
//if motorSpeed > 0, set the speed
if(motorSpeed > 0) {
analogWrite(s3A, motorSpeed);
} else {
//stop the motor
digitalWrite(s3A, LOW);
}
}
Need More Satellite I/O? Checkout the new Port Extender Satellite board.