Overview
This tutorial will teach you how to use PWM Select Jumpers with a Servo Motor.
Components
Arduino
A/B USB cable
Museduino Shield
Museduino Smorgasboard
CAT5 cable
servo motor
screw driver
prototyping wire
Setup
Before we get started, let’s learn about the PWM Select feature on the Main Shield. Locate the header pins (2×3 block) labeled “PWM” between the RJ-45 connecters.
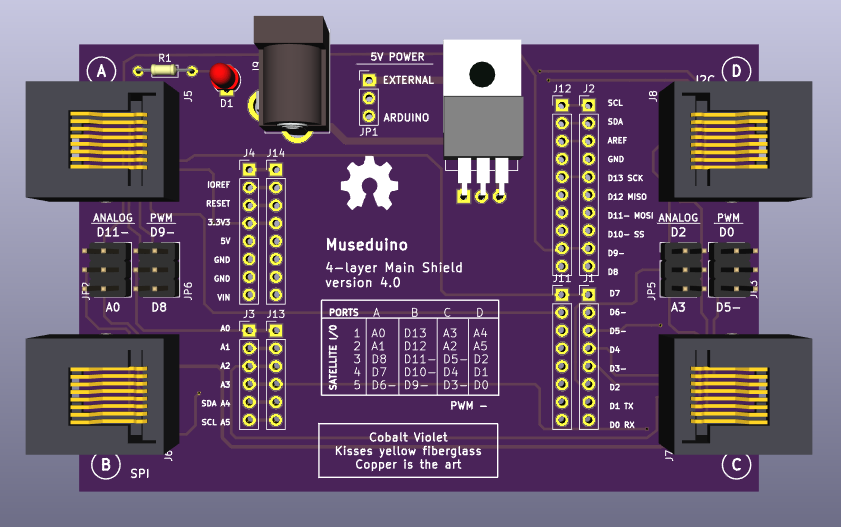
PWM Select feature is a double-pole, double-throw (DPDT) switch. Learn more about DPDT switches here: https://learn.sparkfun.com/tutorials/switch-basics/all
The switches on the main shield are designed to swap pins between two ports. The PWM switch on the left of the main shield can be used to swap Satellite I/O pin 3 on Port A with Satellite I/O pin 5 on B. The PWM switch on the right of the main shield can be used to swap Satellite Pin 3 on Port C with Satellite I/O pin 5 on D.
In order to use the switches, you must use the jumper shunts provided. There are two jumper shunts for each PWM switch (2×3 block) and they both must be placed in an Up or Down position.
Below is a chart to assist with the proper jumper configuration:
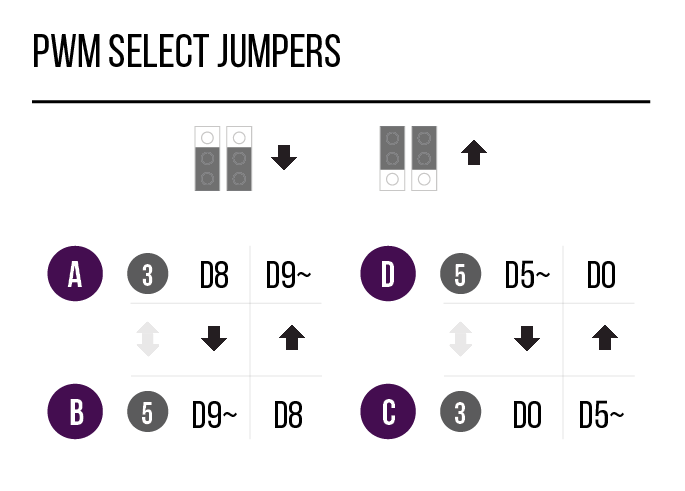
Now that you have learned about the PWM Select feature, let’s implement a servo motor on Arduino Pin D5~.
The chart shows that Arduino Pin D5~ is available from Satellite I/O pin 3 on Port C. Since the PWM jumpers can be used to swap Satellite I/O pin 3 on Port C with Satellite I/O pin 5 on D, this means we can swap D5~ and D0. Place the jumper shunts in a “Down” position to make D5~ available from Satellite Pin 5 on Port D.
Next, connect a servo motor motor to Satellite I/O pin 5. We will need to use Power (screw terminal 1), Signal (screw terminal 3), Ground (screw terminal 4) on either a Smorgasboard or External Power Board.
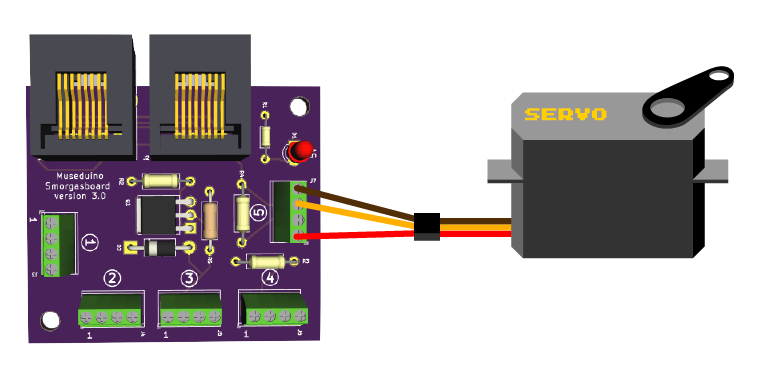
Now connect the Smorgasboard to Port D on the main shield.
Code
Cop code below or download from Github.
Upload the sketch. Make sure your PWM jumpers are in the correct position.
/*
Museduino | Servo Motors (PWM Select) Tutorial
Servo Motor repeatedly turns from 0 to 180 degrees, then 180 to 0 degrees.
*/
#include
Servo servo; // create servo object to control a servo
// a maximum of eight servo objects can be created
int pos = 0; // variable to store the servo position
//Use PWM Select Jumpers to switch Arduino pin D0 with D5~
int s5D = 5; // Arduino Pin D5~ - Satellite I/O Pin 5 on Port D
void setup()
{
servo.attach(s5D); // attaches the servo on pin D5~ to the servo object
}
void loop()
{
for (pos = 0; pos <= 180; pos += 1) { // goes from 0 degrees to 180 degrees
// in steps of 1 degree
servo.write(pos); // tell servo to go to position in variable 'pos'
delay(15); // waits 15ms for the servo to reach the position
}
for (pos = 180; pos >= 0; pos -= 1) { // goes from 180 degrees to 0 degrees
servo.write(pos); // tell servo to go to position in variable 'pos'
delay(15); // waits 15ms for the servo to reach the position
}
}
Want to control the servo using a potentiometer?